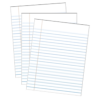
exampleB1.cc
Storyboard
```
#include 'B1DetectorConstruction.hh'
#include 'B1ActionInitialization.hh'
#ifdef G4MULTITHREADED
#include 'G4MTRunManager.hh'
#else
#include 'G4RunManager.hh'
#endif
#include 'G4UImanager.hh'
#include 'QBBC.hh'
#include 'G4VisExecutive.hh'
#include 'G4UIExecutive.hh'
#include 'Randomize.hh'
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
int main(int argc,char** argv)
{
// Detect interactive mode (if no arguments) and define UI session
//
G4UIExecutive* ui = 0;
if ( argc == 1 ) {
ui = new G4UIExecutive(argc, argv);
}
// Choose the Random engine
G4Random::setTheEngine(new CLHEP::RanecuEngine);
// Construct the default run manager
//
#ifdef G4MULTITHREADED
G4MTRunManager* runManager = new G4MTRunManager;
#else
G4RunManager* runManager = new G4RunManager;
#endif
// Set mandatory initialization classes
//
// Detector construction
runManager->SetUserInitialization(new B1DetectorConstruction());
// Physics list
G4VModularPhysicsList* physicsList = new QBBC;
physicsList->SetVerboseLevel(1);
runManager->SetUserInitialization(physicsList);
// User action initialization
runManager->SetUserInitialization(new B1ActionInitialization());
// Initialize visualization
//
G4VisManager* visManager = new G4VisExecutive;
// G4VisExecutive can take a verbosity argument - see /vis/verbose guidance.
// G4VisManager* visManager = new G4VisExecutive('Quiet');
visManager->Initialize();
// Get the pointer to the User Interface manager
G4UImanager* UImanager = G4UImanager::GetUIpointer();
// Process macro or start UI session
//
if ( ! ui ) {
// batch mode
G4String command = '/control/execute ';
G4String fileName = argv[1];
UImanager->ApplyCommand(command+fileName);
}
else {
// interactive mode
UImanager->ApplyCommand('/control/execute init_vis.mac');
ui->SessionStart();
delete ui;
}
// Job termination
// Free the store: user actions, physics_list and detector_description are
// owned and deleted by the run manager, so they should not be deleted
// in the main() program !
delete visManager;
delete runManager;
}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo.....
```
ID:(9435, 0)
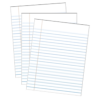
B1ActionInitialization.cc
Storyboard
```
#include 'B1ActionInitialization.hh'
#include 'B1PrimaryGeneratorAction.hh'
#include 'B1RunAction.hh'
#include 'B1EventAction.hh'
#include 'B1SteppingAction.hh'
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
B1ActionInitialization::B1ActionInitialization()
: G4VUserActionInitialization()
{}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
B1ActionInitialization::~B1ActionInitialization()
{}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
void B1ActionInitialization::BuildForMaster() const
{
B1RunAction* runAction = new B1RunAction;
SetUserAction(runAction);
}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
void B1ActionInitialization::Build() const
{
SetUserAction(new B1PrimaryGeneratorAction);
B1RunAction* runAction = new B1RunAction;
SetUserAction(runAction);
B1EventAction* eventAction = new B1EventAction(runAction);
SetUserAction(eventAction);
SetUserAction(new B1SteppingAction(eventAction));
}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
```
ID:(9429, 0)
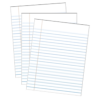
B1DetectorConstruction.cc
Storyboard
```
#include 'B1DetectorConstruction.hh'
#include 'G4RunManager.hh'
#include 'G4NistManager.hh'
#include 'G4Box.hh'
#include 'G4Cons.hh'
#include 'G4Orb.hh'
#include 'G4Sphere.hh'
#include 'G4Trd.hh'
#include 'G4LogicalVolume.hh'
#include 'G4PVPlacement.hh'
#include 'G4SystemOfUnits.hh'
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
B1DetectorConstruction::B1DetectorConstruction()
: G4VUserDetectorConstruction(),
fScoringVolume(0)
{ }
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
B1DetectorConstruction::~B1DetectorConstruction()
{ }
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
G4VPhysicalVolume* B1DetectorConstruction::Construct()
{
// Get nist material manager
G4NistManager* nist = G4NistManager::Instance();
// Envelope parameters
//
G4double env_sizeXY = 20*cm, env_sizeZ = 30*cm;
G4Material* env_mat = nist->FindOrBuildMaterial('G4_WATER');
// Option to switch on/off checking of volumes overlaps
//
G4bool checkOverlaps = true;
//
// World
//
G4double world_sizeXY = 1.2*env_sizeXY;
G4double world_sizeZ = 1.2*env_sizeZ;
G4Material* world_mat = nist->FindOrBuildMaterial('G4_AIR');
G4Box* solidWorld =
new G4Box('World', //its name
0.5*world_sizeXY, 0.5*world_sizeXY, 0.5*world_sizeZ); //its size
G4LogicalVolume* logicWorld =
new G4LogicalVolume(solidWorld, //its solid
world_mat, //its material
'World'); //its name
G4VPhysicalVolume* physWorld =
new G4PVPlacement(0, //no rotation
G4ThreeVector(), //at (0,0,0)
logicWorld, //its logical volume
'World', //its name
0, //its mother volume
false, //no boolean operation
0, //copy number
checkOverlaps); //overlaps checking
//
// Envelope
//
G4Box* solidEnv =
new G4Box('Envelope', //its name
0.5*env_sizeXY, 0.5*env_sizeXY, 0.5*env_sizeZ); //its size
G4LogicalVolume* logicEnv =
new G4LogicalVolume(solidEnv, //its solid
env_mat, //its material
'Envelope'); //its name
new G4PVPlacement(0, //no rotation
G4ThreeVector(), //at (0,0,0)
logicEnv, //its logical volume
'Envelope', //its name
logicWorld, //its mother volume
false, //no boolean operation
0, //copy number
checkOverlaps); //overlaps checking
//
// Shape 1
//
G4Material* shape1_mat = nist->FindOrBuildMaterial('G4_A-150_TISSUE');
G4ThreeVector pos1 = G4ThreeVector(0, 2*cm, -7*cm);
// Conical section shape
G4double shape1_rmina = 0.*cm, shape1_rmaxa = 2.*cm;
G4double shape1_rminb = 0.*cm, shape1_rmaxb = 4.*cm;
G4double shape1_hz = 3.*cm;
G4double shape1_phimin = 0.*deg, shape1_phimax = 360.*deg;
G4Cons* solidShape1 =
new G4Cons('Shape1',
shape1_rmina, shape1_rmaxa, shape1_rminb, shape1_rmaxb, shape1_hz,
shape1_phimin, shape1_phimax);
G4LogicalVolume* logicShape1 =
new G4LogicalVolume(solidShape1, //its solid
shape1_mat, //its material
'Shape1'); //its name
new G4PVPlacement(0, //no rotation
pos1, //at position
logicShape1, //its logical volume
'Shape1', //its name
logicEnv, //its mother volume
false, //no boolean operation
0, //copy number
checkOverlaps); //overlaps checking
//
// Shape 2
//
G4Material* shape2_mat = nist->FindOrBuildMaterial('G4_BONE_COMPACT_ICRU');
G4ThreeVector pos2 = G4ThreeVector(0, -1*cm, 7*cm);
// Trapezoid shape
G4double shape2_dxa = 12*cm, shape2_dxb = 12*cm;
G4double shape2_dya = 10*cm, shape2_dyb = 16*cm;
G4double shape2_dz = 6*cm;
G4Trd* solidShape2 =
new G4Trd('Shape2', //its name
0.5*shape2_dxa, 0.5*shape2_dxb,
0.5*shape2_dya, 0.5*shape2_dyb, 0.5*shape2_dz); //its size
G4LogicalVolume* logicShape2 =
new G4LogicalVolume(solidShape2, //its solid
shape2_mat, //its material
'Shape2'); //its name
new G4PVPlacement(0, //no rotation
pos2, //at position
logicShape2, //its logical volume
'Shape2', //its name
logicEnv, //its mother volume
false, //no boolean operation
0, //copy number
checkOverlaps); //overlaps checking
// Set Shape2 as scoring volume
//
fScoringVolume = logicShape2;
//
//always return the physical World
//
return physWorld;
}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
```
ID:(9430, 0)
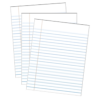
B1EventAction.cc
Storyboard
```
#include 'B1EventAction.hh'
#include 'B1RunAction.hh'
#include 'G4Event.hh'
#include 'G4RunManager.hh'
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
B1EventAction::B1EventAction(B1RunAction* runAction)
: G4UserEventAction(),
fRunAction(runAction),
fEdep(0.)
{}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
B1EventAction::~B1EventAction()
{}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
void B1EventAction::BeginOfEventAction(const G4Event*)
{
fEdep = 0.;
}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
void B1EventAction::EndOfEventAction(const G4Event*)
{
// accumulate statistics in run action
fRunAction->AddEdep(fEdep);
}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
```
ID:(9431, 0)
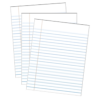
B1PrimaryGeneratorAction.cc
Storyboard
```
#include 'B1PrimaryGeneratorAction.hh'
#include 'G4LogicalVolumeStore.hh'
#include 'G4LogicalVolume.hh'
#include 'G4Box.hh'
#include 'G4RunManager.hh'
#include 'G4ParticleGun.hh'
#include 'G4ParticleTable.hh'
#include 'G4ParticleDefinition.hh'
#include 'G4SystemOfUnits.hh'
#include 'Randomize.hh'
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
B1PrimaryGeneratorAction::B1PrimaryGeneratorAction()
: G4VUserPrimaryGeneratorAction(),
fParticleGun(0),
fEnvelopeBox(0)
{
G4int n_particle = 1;
fParticleGun = new G4ParticleGun(n_particle);
// default particle kinematic
G4ParticleTable* particleTable = G4ParticleTable::GetParticleTable();
G4String particleName;
G4ParticleDefinition* particle
= particleTable->FindParticle(particleName='gamma');
fParticleGun->SetParticleDefinition(particle);
fParticleGun->SetParticleMomentumDirection(G4ThreeVector(0.,0.,1.));
fParticleGun->SetParticleEnergy(6.*MeV);
}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
B1PrimaryGeneratorAction::~B1PrimaryGeneratorAction()
{
delete fParticleGun;
}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
void B1PrimaryGeneratorAction::GeneratePrimaries(G4Event* anEvent)
{
//this function is called at the begining of ecah event
//
// In order to avoid dependence of PrimaryGeneratorAction
// on DetectorConstruction class we get Envelope volume
// from G4LogicalVolumeStore.
G4double envSizeXY = 0;
G4double envSizeZ = 0;
if (!fEnvelopeBox)
{
G4LogicalVolume* envLV
= G4LogicalVolumeStore::GetInstance()->GetVolume('Envelope');
if ( envLV ) fEnvelopeBox = dynamic_cast
}
if ( fEnvelopeBox ) {
envSizeXY = fEnvelopeBox->GetXHalfLength()*2.;
envSizeZ = fEnvelopeBox->GetZHalfLength()*2.;
}
else {
G4ExceptionDescription msg;
msg << 'Envelope volume of box shape not found.
';
msg << 'Perhaps you have changed geometry.
';
msg << 'The gun will be place at the center.';
G4Exception('B1PrimaryGeneratorAction::GeneratePrimaries()',
'MyCode0002',JustWarning,msg);
}
G4double size = 0.8;
G4double x0 = size * envSizeXY * (G4UniformRand()-0.5);
G4double y0 = size * envSizeXY * (G4UniformRand()-0.5);
G4double z0 = -0.5 * envSizeZ;
fParticleGun->SetParticlePosition(G4ThreeVector(x0,y0,z0));
fParticleGun->GeneratePrimaryVertex(anEvent);
}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
```
ID:(9432, 0)
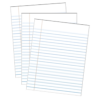
B1RunAction.cc
Storyboard
```
#include 'B1RunAction.hh'
#include 'B1PrimaryGeneratorAction.hh'
#include 'B1DetectorConstruction.hh'
// #include 'B1Run.hh'
#include 'G4RunManager.hh'
#include 'G4Run.hh'
#include 'G4AccumulableManager.hh'
#include 'G4LogicalVolumeStore.hh'
#include 'G4LogicalVolume.hh'
#include 'G4UnitsTable.hh'
#include 'G4SystemOfUnits.hh'
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
B1RunAction::B1RunAction()
: G4UserRunAction(),
fEdep(0.),
fEdep2(0.)
{
// add new units for dose
//
const G4double milligray = 1.e-3*gray;
const G4double microgray = 1.e-6*gray;
const G4double nanogray = 1.e-9*gray;
const G4double picogray = 1.e-12*gray;
new G4UnitDefinition('milligray', 'milliGy' , 'Dose', milligray);
new G4UnitDefinition('microgray', 'microGy' , 'Dose', microgray);
new G4UnitDefinition('nanogray' , 'nanoGy' , 'Dose', nanogray);
new G4UnitDefinition('picogray' , 'picoGy' , 'Dose', picogray);
// Register accumulable to the accumulable manager
G4AccumulableManager* accumulableManager = G4AccumulableManager::Instance();
accumulableManager->RegisterAccumulable(fEdep);
accumulableManager->RegisterAccumulable(fEdep2);
}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
B1RunAction::~B1RunAction()
{}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
void B1RunAction::BeginOfRunAction(const G4Run*)
{
// inform the runManager to save random number seed
G4RunManager::GetRunManager()->SetRandomNumberStore(false);
// reset accumulables to their initial values
G4AccumulableManager* accumulableManager = G4AccumulableManager::Instance();
accumulableManager->Reset();
}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
void B1RunAction::EndOfRunAction(const G4Run* run)
{
G4int nofEvents = run->GetNumberOfEvent();
if (nofEvents == 0) return;
// Merge accumulables
G4AccumulableManager* accumulableManager = G4AccumulableManager::Instance();
accumulableManager->Merge();
// Compute dose = total energy deposit in a run and its variance
//
G4double edep = fEdep.GetValue();
G4double edep2 = fEdep2.GetValue();
G4double rms = edep2 - edep*edep/nofEvents;
if (rms > 0.) rms = std::sqrt(rms); else rms = 0.;
const B1DetectorConstruction* detectorConstruction
= static_cast
(G4RunManager::GetRunManager()->GetUserDetectorConstruction());
G4double mass = detectorConstruction->GetScoringVolume()->GetMass();
G4double dose = edep/mass;
G4double rmsDose = rms/mass;
// Run conditions
// note: There is no primary generator action object for 'master'
// run manager for multi-threaded mode.
const B1PrimaryGeneratorAction* generatorAction
= static_cast
(G4RunManager::GetRunManager()->GetUserPrimaryGeneratorAction());
G4String runCondition;
if (generatorAction)
{
const G4ParticleGun* particleGun = generatorAction->GetParticleGun();
runCondition += particleGun->GetParticleDefinition()->GetParticleName();
runCondition += ' of ';
G4double particleEnergy = particleGun->GetParticleEnergy();
runCondition += G4BestUnit(particleEnergy,'Energy');
}
//
if (IsMaster()) {
G4cout
<< G4endl
<< '--------------------End of Global Run-----------------------';
}
else {
G4cout
<< G4endl
<< '--------------------End of Local Run------------------------';
}
G4cout
<< G4endl
<< ' The run consists of ' << nofEvents << ' '<< runCondition
<< G4endl
<< ' Cumulated dose per run, in scoring volume : '
<< G4BestUnit(dose,'Dose') << ' rms = ' << G4BestUnit(rmsDose,'Dose')
<< G4endl
<< '------------------------------------------------------------'
<< G4endl
<< G4endl;
}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
void B1RunAction::AddEdep(G4double edep)
{
fEdep += edep;
fEdep2 += edep*edep;
}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
```
ID:(9433, 0)
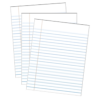
B1SteppingAction.cc
Storyboard
```
#include 'B1SteppingAction.hh'
#include 'B1EventAction.hh'
#include 'B1DetectorConstruction.hh'
#include 'G4Step.hh'
#include 'G4Event.hh'
#include 'G4RunManager.hh'
#include 'G4LogicalVolume.hh'
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
B1SteppingAction::B1SteppingAction(B1EventAction* eventAction)
: G4UserSteppingAction(),
fEventAction(eventAction),
fScoringVolume(0)
{}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
B1SteppingAction::~B1SteppingAction()
{}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
void B1SteppingAction::UserSteppingAction(const G4Step* step)
{
if (!fScoringVolume) {
const B1DetectorConstruction* detectorConstruction
= static_cast
(G4RunManager::GetRunManager()->GetUserDetectorConstruction());
fScoringVolume = detectorConstruction->GetScoringVolume();
}
// get volume of the current step
G4LogicalVolume* volume
= step->GetPreStepPoint()->GetTouchableHandle()
->GetVolume()->GetLogicalVolume();
// check if we are in scoring volume
if (volume != fScoringVolume) return;
// collect energy deposited in this step
G4double edepStep = step->GetTotalEnergyDeposit();
fEventAction->AddEdep(edepStep);
}
//....oooOO0OOooo........oooOO0OOooo........oooOO0OOooo........oooOO0OOooo......
```
ID:(9434, 0)